Golang Integer to String
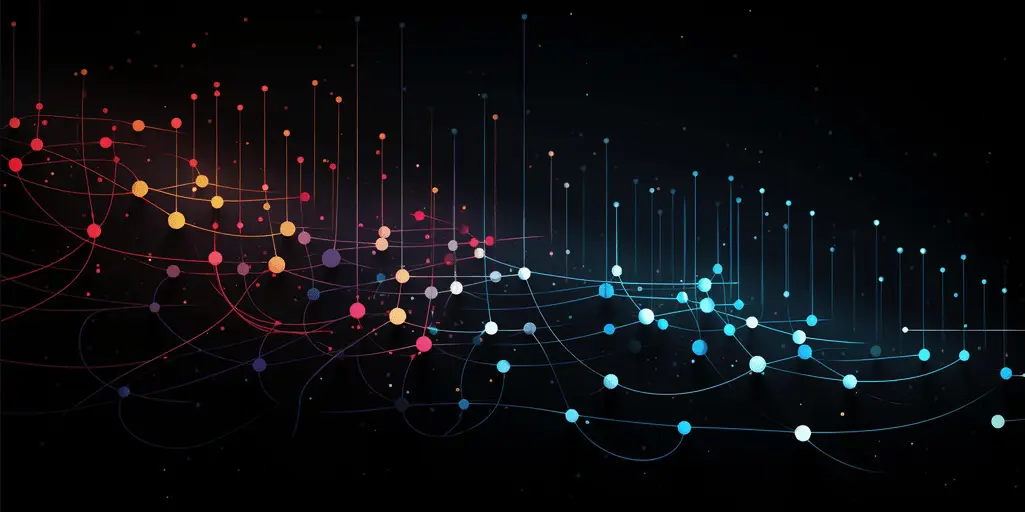
The Go standard library offers the strconv
package which has two functions to convert an integer to a string.
In the following, let’s go into detail on how and where to use which one.
strconv.Itoa(i int)
The strconv.Itoa(i int)
function converts an integer to a string. The function name is short for “integer to ASCII”.
The function takes an integer as an argument and returns a string. Internally, it is calling the FormatInt
function with base 10.
package main
import (
"fmt"
"strconv"
)
func main() {
i := 42
s := strconv.Itoa(i)
fmt.Printf("%T %v\n", s, s)
}
The output of the above program is:
string 42
strconv.FormatInt(i int64, base int)
The strconv.FormatInt(i int64, base int)
function converts an integer to a string.
It takes two arguments, the integer to convert and the base to use for the conversion.
The base must be between 2 and 36, inclusive. It’s input integer requires type int64
, conversion must be done explicitly.
package main
import (
"fmt"
"strconv"
)
func main() {
i := 42
s := strconv.FormatInt(int64(i), 10)
sHex := strconv.FormatInt(int64(i), 16)
fmt.Printf("%T %v\n", s, s)
fmt.Printf("%T %v\n", sHex, sHex)
}
The output of the above program is:
string 42
string 2a
Conclusion
In conclusion, the strconv.Itoa(i int)
function is the easiest way to convert an integer to a string.
If you need more control over the conversion, use the strconv.FormatInt(i int64, base int)
function.